Overview
In many student clubs or even in the corporate world, there are many instances in which the organisation needs to match interviewers with interviewees, especially during times of mass recruitment. Scheduler is a desktop application that was conceived to automate this laborious process of scheduling interviews and to minimise human errors in the scheduing process.
The main feature of Scheduler is to match interviewees with the available time slots of interviewers, so as to generate an overall interview timetable for all interviewees and interviewers. The user is able to interact with the application using a command-line interface (CLI), and it also comes with a graphical user interface (GUI) created using JavaFX. It is written in Java and has about 10 kLoC.
Summary of contributions
-
Major enhancement: Added the ability to automatically generate email
-
What it does: It allows the user to automatically generate the email to be used for sending to an interviewee after the interview scheduling process has been completed. The email will be populated with information such as the allocated time slot and another content that can be configured as part of the user preferences.
-
Justification: This feature drastically improves the efficiency of the user as it is extremely tedious to extract out each interviewee’s email and allocated time slot and manually crafting a unique message to each interviewee after the interview allocation is completed.
-
Highlights: A significant portion of the Storage to disk had to be redesigned in order to accommodate the deviation of the program’s original architecture of being an Address Book into something that is able to hold different categories of people.
-
Credits: None
-
-
Minor enhancement: Restructured the program’s Model and Storage to accommodate the change in the program’s architecture and integrated with changes from other aspects of the program.
-
Code contributed: Functional & Test code
-
Other contributions:
-
Project management:
-
Ensuring that the team is keeping up with weekly progress to ensure almost 100% of the tasks for each milestone is hit.
-
Managed the allocation of issues and PRs to corresponding milestones and the triaging of issues reported.
-
Necessary general code enhancements on renaming the product from AddressBook to Scheduler (Pull requests #104 and #159).
-
-
Enhancements to existing features:
-
Expanded the functionality of the user preferences storage to allow for the customisation of the email message body sent to interviewees (Pull request #107).
-
Developed unit and integration tests for various aspects of the codebase to bring the code coverage from 70% to 80%. (Pull requests #158 #168 #172 #180 #184).
-
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Sending of email email
Constraints
-
A supported mail client should be installed on your machine. This mail client should be able to handle the
mailto:
URI scheme. You can open your web browser and typemailto:test@example.com
to verify if your machine has a supported mail client installed. -
In the software, an email is considered to be sent if the email dialog has successfully appeared on your screen. It does not recognise whether or not you have actually sent the email across the Internet successfully.
-
For all command types, the
schedule
command must be run first, as an email dialog will only appear for interviewees that have slots allocated to them.
Sending of interview time slot to interviewee
Opens an email dialog containing the interviewee’s allocated interview time slot to a particular interviewee specified, including other details such as the interviewer and location.
Format: email ct/timeslot n/INTERVIEWEE NAME
Notes:
-
The email dialog will only appear if the interviewee’s email is present in the database and that the interview schedule has already been generated.
-
The email dialog will appear even if the interviewee has been emailed before.
-
The email dialog will also not appear if you do not have a supported mail client installed on your computer.
Sending of interview time slot to all interviewees
Opens an email dialog containing the interviewee’s allocated interview time slot to every interviewee, including other details such as the interviewer and location. A summary report is then generated at the end of the command execution.
Format: email ct/alltimeslot
Notes:
-
The email dialog will only appear if the interviewee’s email is present in the database and that the interview schedule has already been generated.
-
The email dialog will not appear if the interviewee has been emailed previously.
-
The email dialog will also not appear if you do not have a supported mail client installed on your computer.
Checking status of emails sent
Provides a summary report of the number of interviewees with emails sent and the total number of interviewees stored in the database.
Format: email ct/status
Sending of interview results to an interviewee [Coming in v2.0]
Opens an email dialog containing the interviewee’s result/interview outcome and other details that you might want to include.
Format: email ct/results n/INTERVIEWEE NAME
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Storage component
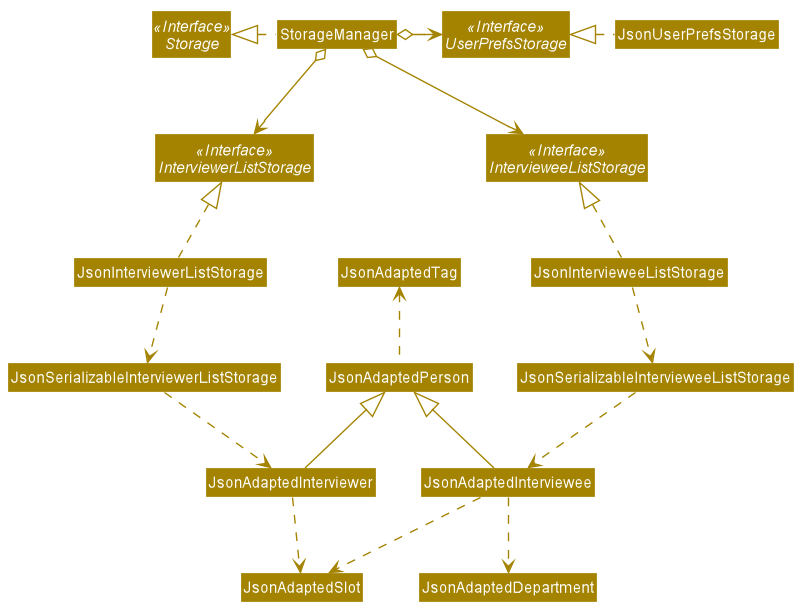
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the list of Interviewees data in json format and read it back.
-
can save the list of Interviewers data in json format and read it back.
Implementation
The Email feature makes use of the java.awt.Desktop
package to activate the default Mail client of the user. For the case of computers without a Mail client available, this feature will not be supported (mainly for headless computers such as servers).
-
The
To:
field is automatically populated with all the emails that are tagged to a particular Interviewee. This combines all the emails of both thePERSONAL
andNUS
email types. -
The
Cc:
field is configurable by the user via an optional user preferences file (located inpreferences.json
) -
The subject and message body are also automatically populated with details that are relevant to the Interviewee, depending on the context of the command used.
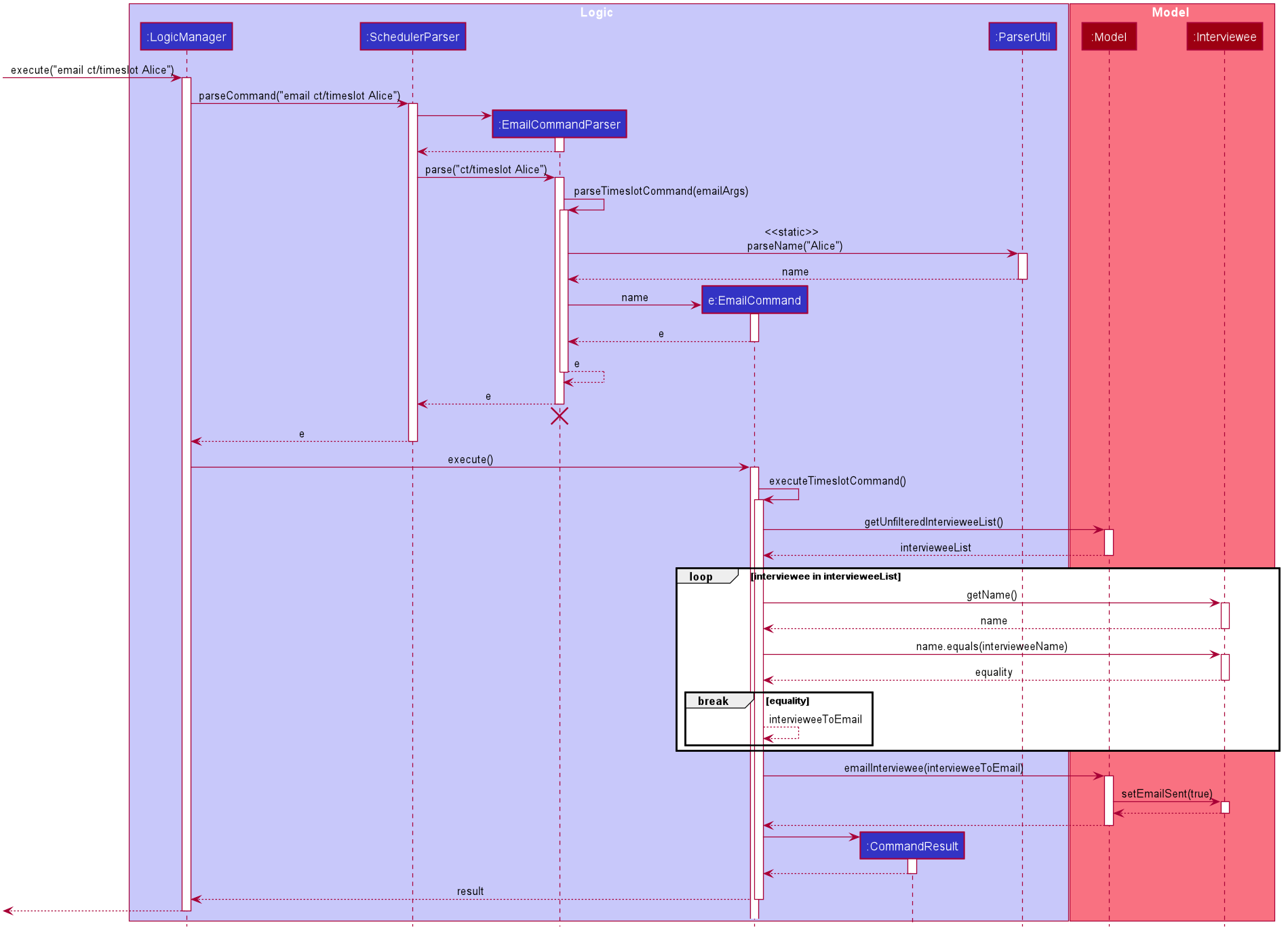
A similar implementation is used when emailing all interviewees (i.e. using the alltimeslot
command type). However, interviewees with the emailSent
boolean variable set to true will not be sent an email again. This can be overridden by emailing that particular interviewee again (using the timeslot
command type).
The user is also able to check the email sending status (i.e. if a particular interviewee has been emailed before) using the status
command type. It is simply going through the list of interviewees stored in the application and counting the number of interviewees with the emailSent
boolean variable set to true.
To the application, an email is considered as sent when the email window successfully opens on the user’s computer. It does not recognise the actual email being sent across the Internet. |
Example: Email interview timeslot to an interviewee
When the application opens an email dialog for sending the email to the selected Interviewee, the email dialog will be automatically populated with information on his/her allocated interview timeslot. The email message body also contains information that are customised to each Interviewee.
Details that vary according to the Interviewee include:
-
Name of the Interviewee
-
Date and time of allocated timeslot
Additional details that can be configured by the user (as user preferences) include:
-
Location to report
-
Any other additional information such as dress code.
The message content can also be configured by the user. However, a default template will be used when no such configuration file exists or is provided.
Emailing an Interviewee
-
Import .csv files of interviewers' availability and interviewees' details using the
import
command.-
Please refer to the QuickStart section in the user guide to download the templates of the interviewers' availability and interviewees details.
-
-
Type
schedule
in the text console of the app and press Enter.-
You can use the sample test data below to get a combination of interviewees and interviewers that can be matched.
-
-
After an interview schedule has been successfully generated, you can try to email one of the interviewees that have been allocated a slot using the
email ct/timeslot
command. For the sample test data above, you can tryemail ct/timeslot n/John Doe
to send an email to John Doe.-
Expected: Your mail client will open with the Compose window. The
To
,Cc
,Subject
and message body is pre-populated with the details specific to the interviewee that you have selected.
-
-
If you do not have a mail client installed, or you are running this software in a headless machine, it is expected that no Compose windows will appear, and an error message will be returned about having a missing mail client.
Emailing all Interviewees
-
Import .csv files of interviewers' availability and interviewees' details using the
import
command.-
Please refer to the QuickStart section in the user guide to download the templates of the interviewers' availability and interviewees details.
-
-
Type
schedule
in the text console of the app and press Enter.-
You can use the sample test data below to get a combination of interviewees and interviewers that can be matched.
-
-
After an interview schedule has been successfully generated, email one interviewee using the
email ct/timeslot
command and choosing any interviewee that has a slot allocated. -
After you emailed one of the interviewees, you can try to email the remaining interviewees using the
email ct/alltimeslot
command.-
Expected: Your mail client will open with multiple Compose windows for each of the interviewees that have been allocated a slot but have not been emailed yet. The interviewee that was emailed in step 3 will not appear again.
-
-
If you do not have a mail client installed, or you are running this software in a headless machine, it is expected that no Compose windows will appear, and an error message will be returned about having a missing mail client.
Getting the status of the email sending process
-
Import .csv files of interviewers' availability and interviewees' details using the
import
command.-
Please refer to the QuickStart section in the user guide to download the templates of the interviewers' availability and interviewees details.
-
-
Type
schedule
in the text console of the app and press Enter.-
You can use the sample test data below to get a combination of interviewees and interviewers that can be matched.
-
-
After an interview schedule has been successfully generated, you can obtain the status of the email sending process using the
email ct/status
command.-
Expected: A message will appear with the statistics on the number of interviewees that have been emailed before. There should be 0 interviewees in this case.
-
-
Send an email to an interviewee that has a slot allocated using the
email ct/timeslot
command. -
After sending the email to the interviewee, you can obtain the status of the email sending process again using the
email ct/status
command.-
Expected: The same message will appear as in step 3, except that the message will reflect that there is 1 interviewee that has been sent an email.
-